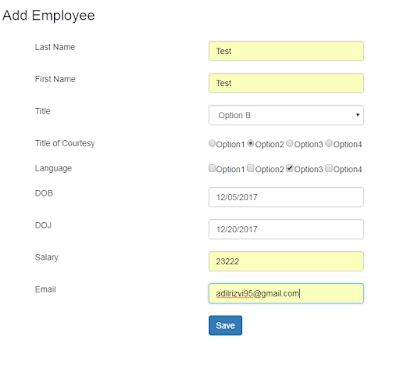
After success of employee addition message is display as in below screen.
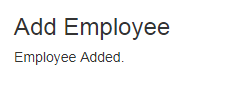
Step 1: Install entity framework, if you don't have it installed already on your computer. Using nuget package manager, is the easiest way to install. A reference to EntityFramework.dll is automatically added.
Open visual studio > Tools > Library Package Manager > Manage NuGet Packages for Solution
Step 2: Add EmployeeContext.cs class file to the Models folder. Add the following "using" declaration (using System.Data.Entity;).
EmployeeContext class derives from DbContext class, and is responsible for establishing a connection to the database. So include connection string in web.config file.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Data.Entity;
namespace MVCDemo.Entity
{
public class EmployeeContext : DbContext
{
public DbSet<Employee> Employees { get; set; }
}
}
Step 3: Add a connection string
<add name="EmployeeContext" connectionString="server=RIZVI-PC\SQLEXPRESS; database=Northworth; integrated security=SSPI" providerName="System.Data.SqlClient" />
Step 4: Map "Employee" model class to the database table, Employees using "Table" and "Key" attribute as shown below.
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.ComponentModel.DataAnnotations.Schema;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace MVCDemo.Entity
{
[Table("Employees")]
public class Employee
{
[Key]
public int EmployeeId { get; set; }
public string LastName { get; set; }
public string FirstName { get; set; }
public string Title { get; set; }
public string TitleofCourtesy { get; set; }
public string Language { get; set; }
public DateTime DOB { get; set; }
public DateTime DOJ { get; set; }
public double Salary { get; set; }
public string Email { get; set; }
}
}
Step 5: Make the changes to action method in controller class.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using MVCDemo.Entity;
using System.Text.RegularExpressions;
namespace MvcDemo.Controllers
{
public class HomeController : Controller
{
public ActionResult Customer()
{
List<SelectListItem> items = new List<SelectListItem>();
items.Add(new SelectListItem
{
Text = "Option1",
Value = "Option1"
});
items.Add(new SelectListItem
{
Text = "Option2",
Value = "Option2"
});
items.Add(new SelectListItem
{
Text = "Option3",
Value = "Option3"
});
items.Add(new SelectListItem
{
Text = "Option4",
Value = "Option4"
});
ViewBag.ListOfItems = items;
TempData["ListofItems"] = items;
return View();
}
[HttpPost]
public ActionResult Customer(Employee objEmployee, string radios, string[] departments)
{
ViewBag.ListOfItems = TempData["ListofItems"];
TempData.Keep();
// Performing server side validation
if (string.IsNullOrEmpty(objEmployee.LastName))
{
ModelState.AddModelError("LastName", "Last Name is required");
}
if (string.IsNullOrEmpty(objEmployee.FirstName))
{
ModelState.AddModelError("FirstName", "First Name is required");
}
if (objEmployee.Title == "0")
{
ModelState.AddModelError("Title", "Title is required");
}
if (string.IsNullOrEmpty(radios))
{
ModelState.AddModelError("TitleofCourtesy", "Title of Coutesy is required");
}
if (departments==null)
{
ModelState.AddModelError("Language", "Language is required");
}
if (!string.IsNullOrEmpty(objEmployee.Email))
{
string emailRegex = @"^([a-zA-Z0-9_\-\.]+)@((\[[0-9]{1,3}" +
@"\.[0-9]{1,3}\.[0-9]{1,3}\.)|(([a-zA-Z0-9\-]+\" +
@".)+))([a-zA-Z]{2,4}|[0-9]{1,3})(\]?)$";
Regex re = new Regex(emailRegex);
if (!re.IsMatch(objEmployee.Email))
{
ModelState.AddModelError("Email", "Email is not valid");
}
}
else
{
ModelState.AddModelError("Email", "Email is required");
}
if (ModelState.IsValid)
{
// Perform Operations
objEmployee.TitleofCourtesy = radios;
// You might have to use string separator like comma or something else.
string dept = "";
foreach (var d in departments)
dept += d.ToString();
objEmployee.Language = dept;
try
{
EmployeeContext db = new EmployeeContext();
db.Employees.Add(objEmployee);
db.SaveChanges();
ViewBag.Message = "Employee Added.";
}
catch (Exception ex)
{
}
}
return View();
}
}
}
In View:
@model MVCDemo.Entity.Customer
@{
Layout = null;
}
<!DOCTYPE html>
<html lang="en">
<head>
<title>Customer</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
</head>
<body>
<div class="container-fluid">
<h3>Add Employee</h3>
@if (ViewBag.Message != null)
{
<h5>@ViewBag.Message</h5>
}
else
{
<div class="container">
@using (Html.BeginForm("Customer", "Home", FormMethod.Post))
{
<div class="clear-fix"> </div>
<div class="row">
<div class="col-md-3">Last Name</div>
<div class="col-md-3">
@Html.TextBoxFor(m => m.LastName, new { id = "txtLastName", @class = "form-control" })
</div>
<div class="col-md-6">
<div class="text-danger" id="errLastName">
@Html.ValidationMessageFor(m => m.LastName)
</div>
</div>
</div>
<div class="clear-fix"> </div>
<div class="row">
<div class="col-md-3">First Name</div>
<div class="col-md-3">
@Html.TextBoxFor(m => m.FirstName, new { id = "txtFirstName", @class = "form-control" })
</div>
<div class="col-md-6">
<div class="text-danger" id="errFirstName">
@Html.ValidationMessageFor(m => m.FirstName)
</div>
</div>
</div>
<div class="clear-fix"> </div>
<div class="row">
<div class="col-md-3">Title</div>
<div class="col-md-3">
@Html.DropDownListFor(m => m.Title,
new List<SelectListItem> {
new SelectListItem { Value = "0" , Text = "Select Title" },
new SelectListItem { Value = "1" , Text = "Option A" },
new SelectListItem { Value = "2" , Text = "Option B" },
new SelectListItem { Value = "3" , Text = "Option C" }
},
new { id = "drpTitle", @class = "form-control" })
</div>
<div class="col-md-6">
<div class="text-danger" id="errTitle">
@Html.ValidationMessageFor(m => m.Title)
</div>
</div>
</div>
<div class="clear-fix"> </div>
<div class="row">
<div class="col-md-3">Title of Courtesy</div>
<div class="col-md-3">
@if (ViewBag.ListOfItems != null)
{
foreach (var c in ViewBag.ListOfItems)
{
<input type="radio" name="radios" value="@c.Value" />@c.Text
}
}
</div>
<div class="col-md-6">
<div class="text-danger" id="errTOC">
@Html.ValidationMessageFor(m => m.TitleofCourtesy)
</div>
</div>
</div>
<div class="clear-fix"> </div>
<div class="row">
<div class="col-md-3">Language</div>
<div class="col-md-3">
@if (ViewBag.ListOfItems != null)
{
foreach (var c in ViewBag.ListOfItems)
{
<input type="checkbox" name="departments" value="@c.Value" />@c.Text
}
}
</div>
<div class="col-md-6">
<div class="text-danger" id="errLanguage">
@Html.ValidationMessageFor(m => m.Language)
</div>
</div>
</div>
<div class="clear-fix"> </div>
<div class="row">
<div class="col-md-3">DOB</div>
<div class="col-md-3">
@Html.TextBoxFor(m => m.DOB, new { id = "txtDOB", @class = "form-control" })
</div>
<div class="col-md-6">
<div class="text-danger" id="errDOB">
@Html.ValidationMessageFor(m => m.DOB)
</div>
</div>
</div>
<div class="clear-fix"> </div>
<div class="row">
<div class="col-md-3">DOJ</div>
<div class="col-md-3">
@Html.TextBoxFor(m => m.DOJ, new { id = "txtDOJ", @class = "form-control" })
</div>
<div class="col-md-6">
<div class="text-danger" id="errDOJ">
@Html.ValidationMessageFor(m => m.DOJ)
</div>
</div>
</div>
<div class="clear-fix"> </div>
<div class="row">
<div class="col-md-3">Salary</div>
<div class="col-md-3">
@Html.TextBoxFor(m => m.Salary, new { id = "txtSalary", @class = "form-control" })
</div>
<div class="col-md-6">
<div class="text-danger hidden" id="errSalary">
@Html.ValidationMessageFor(m => m.Salary)
</div>
</div>
</div>
<div class="clear-fix"> </div>
<div class="row">
<div class="col-md-3">Email</div>
<div class="col-md-3">
@Html.TextBoxFor(m => m.Email, new { id = "txtEmail", @class = "form-control" })
</div>
<div class="col-md-6">
<div class="text-danger" id="errEmail">
@Html.ValidationMessageFor(m => m.Email)
</div>
</div>
</div>
<div class="clear-fix"> </div>
<div class="row">
<div class="col-md-3"> </div>
<div class="col-md-3">
<input type="submit" id="btnSave" class="btn btn-primary" value="Save" />
</div>
<div class="col-md-6">
</div>
</div>
<div class="clear-fix"> </div>
}
</div>
}
</div>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css">
<script src="https://code.jquery.com/jquery-1.12.4.js"></script>
<script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
<script>
$(function () {
$("#txtDOB").datepicker({ maxDate: '0' });
$("#txtDOJ").datepicker({ minDate: '0' });
$("#btnSave").click(function () {
});
});
</script>
</body>
</html>
Step 6: Existing databases do not need, database initializer so it can be turned off so copy and paste the following code in Application_Start() function, in Global.asax file.
Database.SetInitializer<MVCDemo.Models.EmployeeContext>(null);
using System;
using System.Collections.Generic;
using System.Data.Entity;
using System.Linq;
using System.Web;
using System.Web.Http;
using System.Web.Mvc;
using System.Web.Optimization;
using System.Web.Routing;
namespace MvcDemo
{
public class MvcApplication : System.Web.HttpApplication
{
protected void Application_Start()
{
Database.SetInitializer<MVCDemo.Entity.EmployeeContext>(null);
AreaRegistration.RegisterAllAreas();
WebApiConfig.Register(GlobalConfiguration.Configuration);
FilterConfig.RegisterGlobalFilters(GlobalFilters.Filters);
RouteConfig.RegisterRoutes(RouteTable.Routes);
BundleConfig.RegisterBundles(BundleTable.Bundles);
AuthConfig.RegisterAuth();
}
}
}