Create dropdownlist : A dropdownlist in MVC is a collection of SelectListItem objects. Depending on your project requirement you may either hard code the values in code or retrieve them from a database table.
Method 1.)
In Controller Method
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace MvcProject.Controllers
{
public class Home3Controller : Controller
{
public ActionResult Index()
{
//Creating generic list
List<SelectListItem> ObjList = new List<SelectListItem>()
{
new SelectListItem { Text = "Latur", Value = "1" },
new SelectListItem { Text = "Pune", Value = "2" },
new SelectListItem { Text = "Mumbai", Value = "3" },
new SelectListItem { Text = "Delhi", Value = "4" },
};
//Assigning generic list to ViewBag
ViewBag.Locations = ObjList;
return View();
}
}
}
In Index.cshtml view
Mtype
<br />
<select>
@foreach (var m in @ViewBag.Locations)
{
<option value= "+@m.Value+">@m.Text </option>
}
</select>
Method 2.) Binding Dropdownlist With Database In asp.net MVC using stored Procedure
Create Table
CREATE TABLE [dbo].[tblMset](
[MID] [int] IDENTITY(1,1) NOT NULL,
[MName] [varchar](50) NULL,
[Status] [bit] NULL,
CONSTRAINT [PK_tblMset] PRIMARY KEY CLUSTERED
(
[MID] ASC
)WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY]
) ON [PRIMARY]
Create Stored Procedure
Create proc [dbo].[Usp_ReadMsets]
As
Begin
select * from tblMset where Status='true'
End
Add connection string in web.config
<connectionStrings>
<add name="SampleDbEntities1" connectionString="data source=VIKASH-PC\SQLEXPRESS;initial catalog=SampleDb;integrated security=True;" />
</connectionStrings>
</configuration>
Create Controller Method
using System;
using System.Collections.Generic;
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace MvcProject.Controllers
{
public class Home3Controller : Controller
{
public ActionResult Index()
{
string connectionstring = ConfigurationManager.ConnectionStrings["SampleDbEntities1"].ToString();
using (SqlConnection con = new SqlConnection(connectionstring))
{
SqlCommand com = new SqlCommand("Usp_ReadMsets", con);
com.CommandType = CommandType.StoredProcedure;
con.Open();
SqlDataReader reader = com.ExecuteReader();
List<SelectListItem> mset = new List<SelectListItem>();
while (reader.Read())
{
mset.Add(new SelectListItem{
Value=(reader["MID"].ToString()),
Text=reader["MName"].ToString()
});
}
ViewBag.Msets = mset;
}
return View();
}
}
}
Add Index.cshtml View
Mtype
<br />
<select>
@foreach (var m in @ViewBag.Msets)
{
<option value= "+@m.Value+">@m.Text </option>
}
</select>
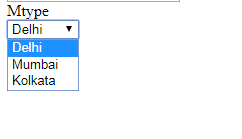
Method 3.) For this example, let's use entity framework to retrieve data and bind dropdownlist.
Add ADO.Net Entity Model in Models folder and add database table. Here name of table is tblMset.
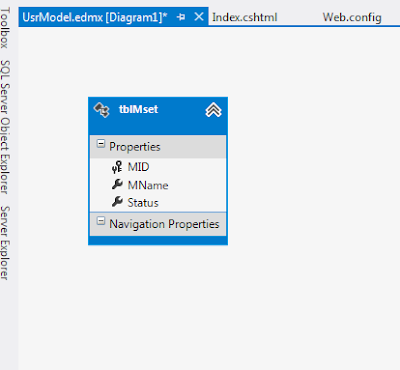
A connection string will be added to web.config file like as given below.
<add name="SampleDbEntities" connectionString="metadata=res://*/Models.UsrModel.csdl|res://*/Models.UsrModel.ssdl|res://*/Models.UsrModel.msl;provider=System.Data.SqlClient;provider connection string="data source=VIKASH-PC\SQLEXPRESS;initial catalog=SampleDb;integrated security=True;MultipleActiveResultSets=True;App=EntityFramework"" providerName="System.Data.EntityClient" /></connectionStrings>
Controller Method
public ActionResult Index()
{
SampleDbEntities db = new SampleDbEntities();
ViewBag.Msets = new SelectList(db.tblMsets,"MID", "MName");
return View();
}
Or Controller Method
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using MvcProject.Models;
namespace MvcProject.Controllers
{
public class Home4Controller : Controller
{
public ActionResult Index()
{
SampleDbEntities db = new SampleDbEntities();
ViewBag.Msets = new SelectList(from x in db.tblMsets where x.Status==true select x, "MID", "MName");
return View();
}
}
}
In Index.cshtml view
Mtype
<br />
<select>
@foreach (var m in @ViewBag.Msets)
{
<option value= "+@m.Value+">@m.Text </option>
}
</select>
Method 1.)
In Controller Method
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace MvcProject.Controllers
{
public class Home3Controller : Controller
{
public ActionResult Index()
{
//Creating generic list
List<SelectListItem> ObjList = new List<SelectListItem>()
{
new SelectListItem { Text = "Latur", Value = "1" },
new SelectListItem { Text = "Pune", Value = "2" },
new SelectListItem { Text = "Mumbai", Value = "3" },
new SelectListItem { Text = "Delhi", Value = "4" },
};
//Assigning generic list to ViewBag
ViewBag.Locations = ObjList;
return View();
}
}
}
In Index.cshtml view
Mtype
<br />
<select>
@foreach (var m in @ViewBag.Locations)
{
<option value= "+@m.Value+">@m.Text </option>
}
</select>
Method 2.) Binding Dropdownlist With Database In asp.net MVC using stored Procedure
Create Table
CREATE TABLE [dbo].[tblMset](
[MID] [int] IDENTITY(1,1) NOT NULL,
[MName] [varchar](50) NULL,
[Status] [bit] NULL,
CONSTRAINT [PK_tblMset] PRIMARY KEY CLUSTERED
(
[MID] ASC
)WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY]
) ON [PRIMARY]
Create Stored Procedure
Create proc [dbo].[Usp_ReadMsets]
As
Begin
select * from tblMset where Status='true'
End
Add connection string in web.config
<connectionStrings>
<add name="SampleDbEntities1" connectionString="data source=VIKASH-PC\SQLEXPRESS;initial catalog=SampleDb;integrated security=True;" />
</connectionStrings>
</configuration>
Create Controller Method
using System;
using System.Collections.Generic;
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace MvcProject.Controllers
{
public class Home3Controller : Controller
{
public ActionResult Index()
{
string connectionstring = ConfigurationManager.ConnectionStrings["SampleDbEntities1"].ToString();
using (SqlConnection con = new SqlConnection(connectionstring))
{
SqlCommand com = new SqlCommand("Usp_ReadMsets", con);
com.CommandType = CommandType.StoredProcedure;
con.Open();
SqlDataReader reader = com.ExecuteReader();
List<SelectListItem> mset = new List<SelectListItem>();
while (reader.Read())
{
mset.Add(new SelectListItem{
Value=(reader["MID"].ToString()),
Text=reader["MName"].ToString()
});
}
ViewBag.Msets = mset;
}
return View();
}
}
}
Add Index.cshtml View
Mtype
<br />
<select>
@foreach (var m in @ViewBag.Msets)
{
<option value= "+@m.Value+">@m.Text </option>
}
</select>
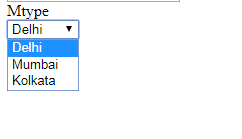
Method 3.) For this example, let's use entity framework to retrieve data and bind dropdownlist.
Add ADO.Net Entity Model in Models folder and add database table. Here name of table is tblMset.
A connection string will be added to web.config file like as given below.
<add name="SampleDbEntities" connectionString="metadata=res://*/Models.UsrModel.csdl|res://*/Models.UsrModel.ssdl|res://*/Models.UsrModel.msl;provider=System.Data.SqlClient;provider connection string="data source=VIKASH-PC\SQLEXPRESS;initial catalog=SampleDb;integrated security=True;MultipleActiveResultSets=True;App=EntityFramework"" providerName="System.Data.EntityClient" /></connectionStrings>
Controller Method
public ActionResult Index()
{
SampleDbEntities db = new SampleDbEntities();
ViewBag.Msets = new SelectList(db.tblMsets,"MID", "MName");
return View();
}
Or Controller Method
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using MvcProject.Models;
namespace MvcProject.Controllers
{
public class Home4Controller : Controller
{
public ActionResult Index()
{
SampleDbEntities db = new SampleDbEntities();
ViewBag.Msets = new SelectList(from x in db.tblMsets where x.Status==true select x, "MID", "MName");
return View();
}
}
}
In Index.cshtml view
Mtype
<br />
<select>
@foreach (var m in @ViewBag.Msets)
{
<option value= "+@m.Value+">@m.Text </option>
}
</select>
No comments:
Post a Comment