What we want to achieve?
In this session we want to simply display all quiz and quiz options (around random 10 quiz) on start quiz screen like as shown below.
- We want to simply display all quiz and quiz options (around random 10 quiz) on start quiz screen.
- We want to simply display quiz and quiz options on start quiz screen one by one with next button to move to next question.(We'll discuss in Next Session)
- We want to display quiz and quiz options on start quiz screen one by one with next and previous button to move to next question or previous question.(We'll discuss in Later Session)
Step 1) We have already created database table for quiz and quiz options management. Lets quickly go through the scripts.
Table Quiz :
CREATE TABLE [dbo].[Quiz](
[Id] [int] IDENTITY(1,1) NOT NULL,
[Question] [varchar](200) NULL,
[AnswerType] [varchar](10) NULL,
PRIMARY KEY CLUSTERED
(
[Id] ASC
)WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY]
) ON [PRIMARY]
Fill this table with sample questions and lets quickly test :
select * from quiz
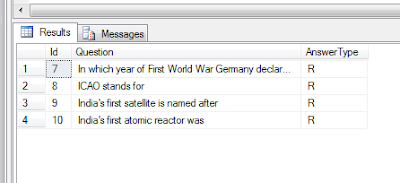
Table QuizOption :
CREATE TABLE [dbo].[QuizOption]( [Id] [int] IDENTITY(1,1) NOT NULL, [Questionid] [varchar](200) NULL, [Option] [varchar](200) NULL, [IsCorrect] [bit] NULL, [AnsType] [varchar](10) NULL, PRIMARY KEY CLUSTERED ( [Id] ASC )WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY] ) ON [PRIMARY]Fill this table with sample quiz options and lets quickly test :select * from quizOption
Step 2) Create Procedures to Read Questions and its options.
Procedure Usp_ReadQuestions :
CREATE PROCEDURE [dbo].[Usp_ReadQuestions]
AS
BEGIN
SELECT TOP(10)* FROM Quiz
ORDER BY NEWID()
END
Procedure Usp_ReadQuizOptionsById :
CREATE PROCEDURE [dbo].[Usp_ReadQuizOptionsById]
@Id int
AS
BEGIN
DECLARE @opts NVARCHAR(MAX);
DECLARE @ids NVARCHAR(MAX);
SELECT
@opts = COALESCE(@opts+'*','') + qo.[Option],
@ids = COALESCE(@ids+'*','') + CAST(qo.[Id] as varchar)
FROM QuizOption qo
WHERE qo.Questionid = @Id ORDER BY qo.[Id]
SELECT @opts AS QuizOptions, @ids OptionIDs
END
Lets quickly test this procedure by passing quiz id 9 and 10 to get its options by star separated:
exec Usp_ReadQuizOptionsById 9
Output:
exec Usp_ReadQuizOptionsById 10
Output:
Step 3) We have already create models for quiz and its options in previous session i.e in part 2. Lets quickly check what we have done and enhanced in SampleMvc.Models :Quiz Model :using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace SampleMvc.Models { public class Quiz { public string Question { get; set; } public int QuestionId { get; set; } public string QuizOptions { get; set; } public string Answertype { get; set; } } }
In QuizOptions Model :
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace SampleMvc.Models { public class QuizOptions { public string Options { get; set; } public string IDs { get; set; } } }
In QuizSet Model : We have created this model to retrieve quiz and all itsoptions to generate quiz set. In this model QuizOptions and OptionIDs willhold star separated quiz options and all its options id. These Options and Ids will be splited and shown there on the screen.using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace SampleMvc.Models { public class QuizSet { public int QuestionId { get; set; } public string Question { get; set; } public string Answertype { get; set; } public string QuizOptions { get; set; } public string OptionIDs { get; set; } } }
Step 4) Create functions to call procedures to Read Questions and its options in SampleMvc.DA.
Note : QuizDA inherits SQLHelper class(check for this class in previous session.)
using System; using System.Collections.Generic; using System.Linq; using System.Text; using SampleMvc.Models; using System.Data.SqlClient; using System.Data; namespace SampleMvc.DA { public class QuizDA : SQLHelper { public List<Quiz> ReadAllQuiz() { try { List<Quiz> questions = new List<Quiz>(); SqlCommand cmd = new SqlCommand(); cmd.CommandText = "Usp_ReadQuestions"; cmd.CommandType = CommandType.StoredProcedure; cmd.Connection = Connection; OpenConnection(); SqlDataReader rdr = cmd.ExecuteReader(); while (rdr.Read()) { Quiz quiz = new Quiz(); quiz.QuestionId = Convert.ToInt32(rdr["Id"]); quiz.Question = rdr["Question"].ToString(); quiz.Answertype = rdr["AnswerType"].ToString(); questions.Add(quiz); } return questions; } catch { throw; } finally { CloseConnection(); } } public QuizOptions ReadQuizOptions(int id) { try { QuizOptions quizoption = new QuizOptions(); DataTable dt = new DataTable(); dt = ExecDataTableProc("Usp_ReadQuizOptionsById", new SqlParameter("@Id", id)); quizoption.Options = dt.Rows[0]["QuizOptions"].ToString(); quizoption.IDs = dt.Rows[0]["OptionIDs"].ToString(); return quizoption; } catch { throw; } finally { CloseConnection(); } } } }
Step 5) Create action methods in controller class. We have created two action methods StartQuiz and ReadAllQuiz.
using SampleMvc.DA;
using SampleMvc.Models;
using System;
using System.Collections.Generic;
using System.Data;
using System.IO;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace SampleMvc.Controllers
{
public class EmployeeTestController : Controller
{
public ActionResult StartQuiz()
{
return View();
}
public JsonResult ReadAllQuiz()
{
List<QuizSet> quizSet = new List<QuizSet>();
QuizDA quizDA = new QuizDA();
List<Quiz> quizs = quizDA.ReadAllQuiz();
foreach (Quiz q in quizs)
{
QuizOptions options = quizDA.ReadQuizOptions(q.QuestionId);
if (options != null)
{
quizSet.Add(new QuizSet
{
QuestionId = q.QuestionId,
Question = q.Question ?? string.Empty,
Answertype=q.Answertype,
QuizOptions = options.Options,
OptionIDs = options.IDs
});
}
}
return Json(quizSet);
}
}
}
Step 6) Create view for action method StartQuiz
@{
Layout = null;
}
<link href="~/Contents/CSS/QuizTemplate.css" rel="stylesheet" />
<link href="~/Contents/W3Css/w3.css" rel="stylesheet" />
<div id="divQuiz" class="displayQuiz w3-container w3-teal">
</div>
<script src="~/Scripts/jquery-3.2.1.js"></script>
<script>
$(document).ready(function () {
displayQuiz();
function displayQuiz() {
$.ajax({
url: '/EmployeeTest/ReadAllQuiz/',
type: 'POST',
contentType: "application/json",
dataType: "json",
success: function (data) {
var count = 1;
if (data.length > 0) {
$.each(data, function (i, q) {
if (q.Answertype == 'R') {
var Opts = q.QuizOptions.split("*");
var OptIds = q.OptionIDs.split("*");
var numOptions = Opts.length;
$(".displayQuiz").append("<h1 style='background-color: lightseagreen;'><div class='quiz'><span class='quizno'>" + (count++) + ".</span><span class='quizTitle'>" + q.Question + "</span></div></h1>");
$(".displayQuiz").append("<ul class='quizOption' id='optionList" + count + "'></ul>")
for (i = 0; i < numOptions; i++) {
$("#optionList" + count).append('<li><input style="width:20px;" type="radio" value=' + OptIds[i] + ' name="dynradio" qid="' + q.QuestionId + '" /><div class="leftOpt";">' + Opts[i] + '</div></li>');
}
}
});
}
else {
}
},
error: function (response) {
console.log(response);
}
});
}
});
</script>
Last Step (Optional) : Set css as per your requirements. Sample is given below :
Use <link rel="stylesheet" href="https://www.w3schools.com/w3css/4/w3.css"> or import it.
In QuizTemplate.css
body {
}
ul {
list-style: none;
}
.leftOpt {
float: right;
width:97%;
}
li {
font-family: 'Roboto', sans-serif;
padding: 10px 0;
clear: both;
}
.quiz {
padding-left: 10px;
}
.quizTitle {
padding-left: 10px;
}
Last Step (Optional) : Set css as per your requirements. Sample is given below :
Use <link rel="stylesheet" href="https://www.w3schools.com/w3css/4/w3.css"> or import it.
In QuizTemplate.css
body {
}
ul {
list-style: none;
}
.leftOpt {
float: right;
width:97%;
}
li {
font-family: 'Roboto', sans-serif;
padding: 10px 0;
clear: both;
}
.quiz {
padding-left: 10px;
}
.quizTitle {
padding-left: 10px;
}
No comments:
Post a Comment